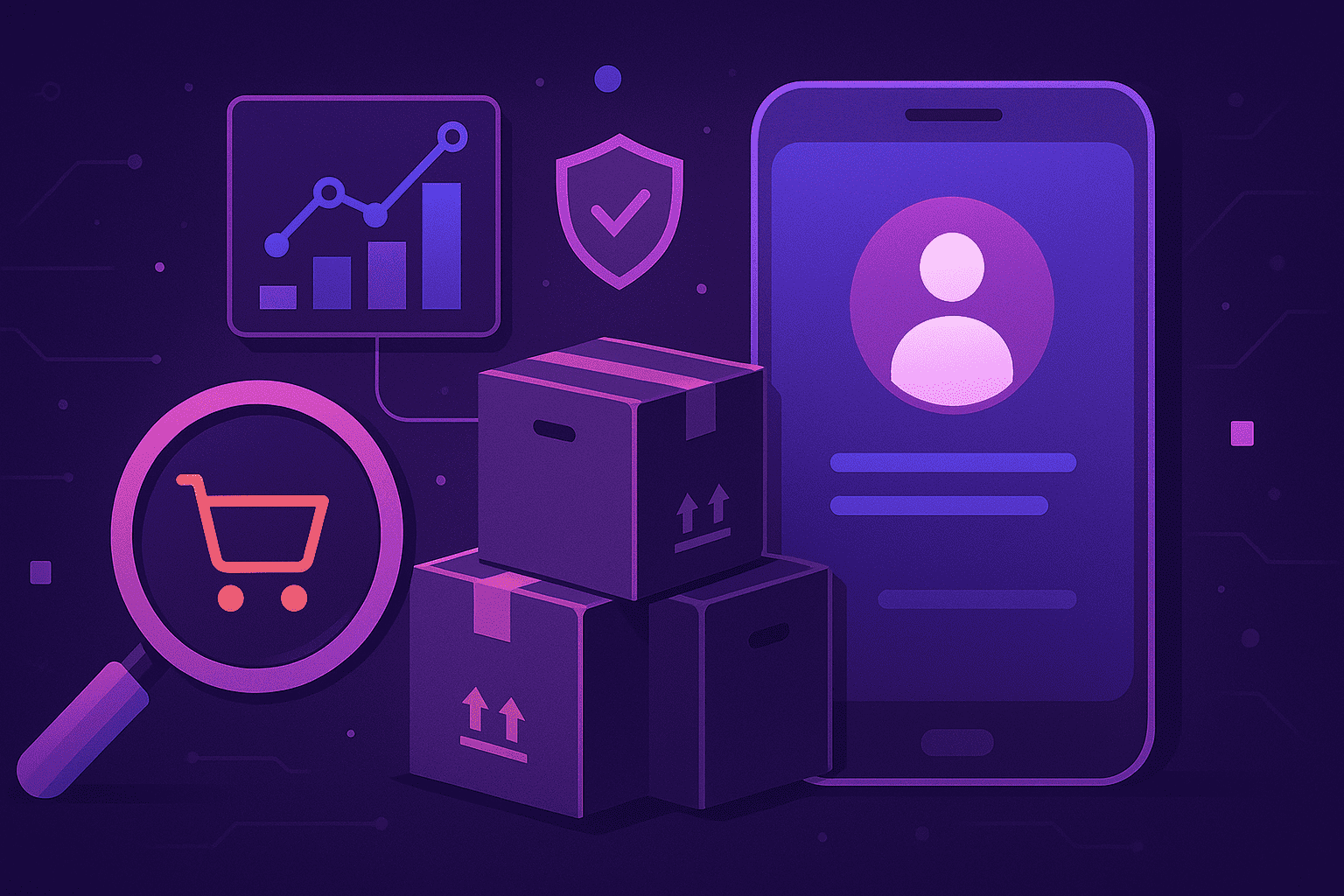
Software bugs are an unavoidable part of software development, and it’s essentially impossible to eliminate all defects from your codebase.
However, despite this disclaimer, you and your developers should still strive to build quality software and eliminate the most disruptive bugs.
Preventing bugs from entering your product in the first place is perhaps the most effective way of doing so.
Although debugging will remove errors from your code, you’ll save time and effort by simply avoiding them from the beginning.
If you’re unsure how exactly to accomplish this, don’t worry—this article will show you how.
Table of Contents
Be clear about product requirements
Before you build software, the first step is to meet up with the product owner, the stakeholders, and your own team to determine the product’s requirements.
This comprehensive and detailed document outlines the software’s features, functionality, behavior, and purpose.
Given the document’s scope, it must be easily understandable.
If anything is ambiguous, developers might make mistakes and build incompatible code that conflicts with the correct functions.
In fact, the number of software bugs resulting from incorrect requirements is startlingly high:
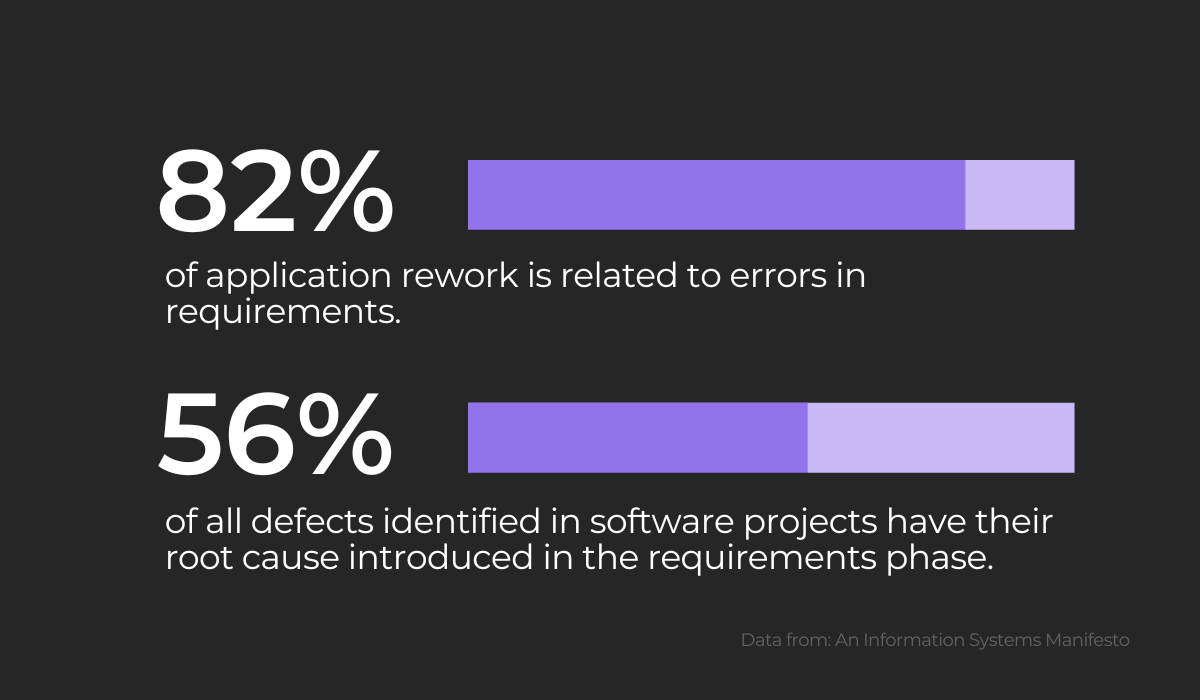
If requirements had been better communicated, many software bugs could have been avoided from the beginning, saving the developers both time and effort.
Furthermore, by prioritizing clarity of communication and quick action, you’re also likely to save on costs.
It’s well known that defects become more expensive to solve the longer they remain in the software development life cycle.
The graph below shows the findings of the IBM System Sciences Institute that illustrate this well:
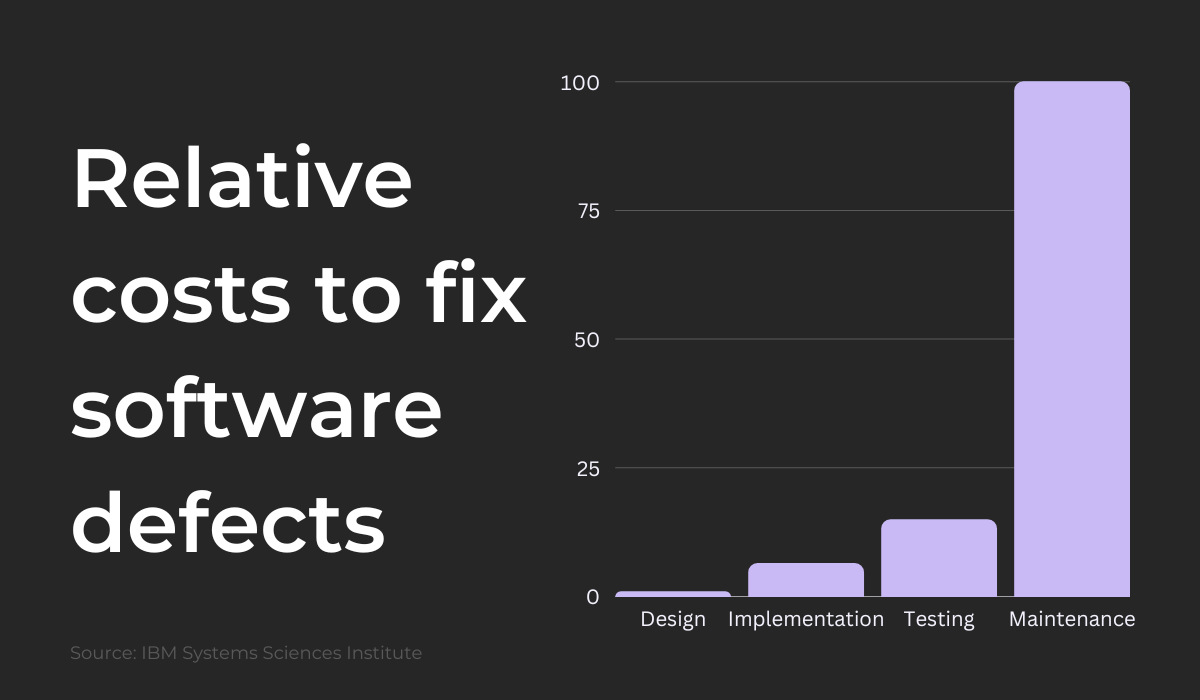
The most economical phase is design—when you can prevent the bugs from appearing in the first place.
Defects are already quite costly during testing and go through the roof when performing maintenance.
However, by pinpointing possible inconsistencies in the requirements document immediately, your bug-related costs will be much lower.

Get unreal data to fix real issues in your app & web.
If you’re unsure how to write clear requirements, INCOSE (the International Council on Systems Engineering) has some guidelines to help craft an easily-understandable document.
Here’s their advice:
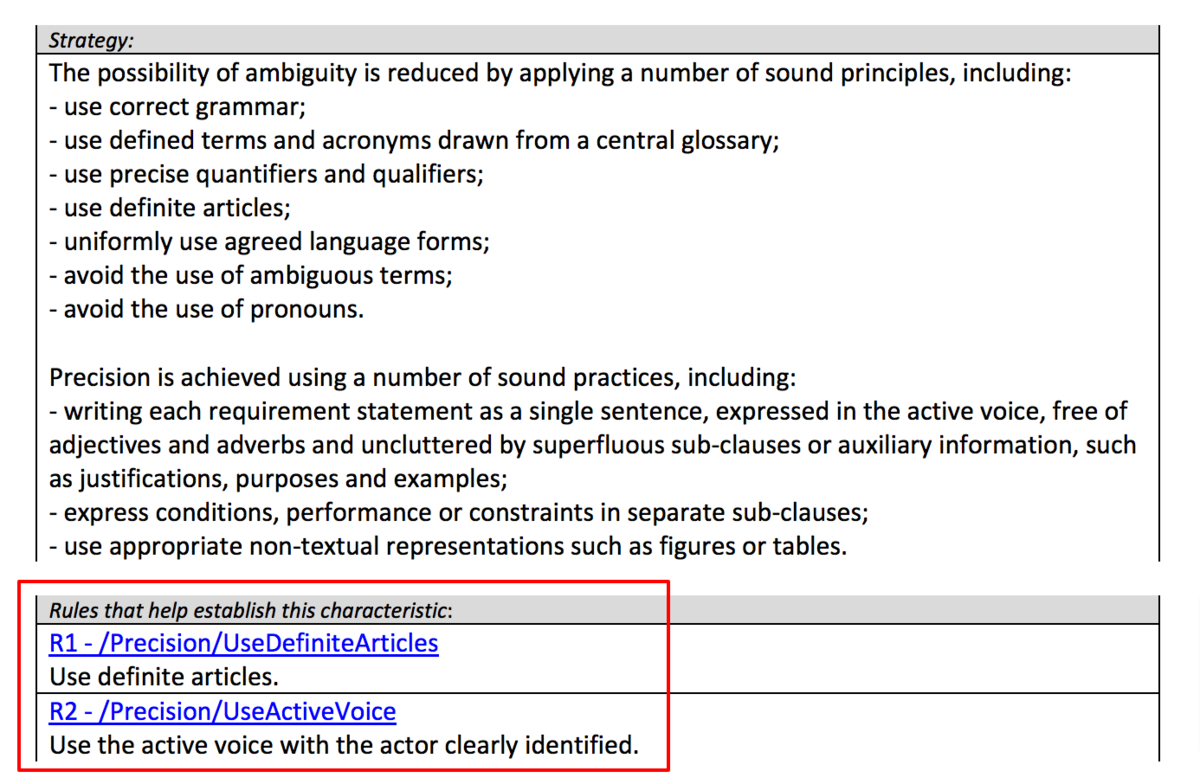
These instructions are applicable to the entire document, including technical specifications, business requirements, and user requirements.
As you can see, they suggest using definite articles to ensure precision, and employing the active voice, since it is frequently much clearer than the passive.
Furthermore, as the text continues, there are an additional 33 rules to help write more comprehensible requirements.
These directions essentially serve as a style guide for your writing.
However, there’s another approach that is possibly even more effective than clear writing—one that doesn’t involve documents whatsoever.
Model-based system engineering is a specification methodology that utilizes domain models instead of natural languages (e.g., English).
Since the specification language is more-or-less purely mathematical, and domain models can be tested, there is a significantly reduced chance of software errors.
Here’s an example of the technique:
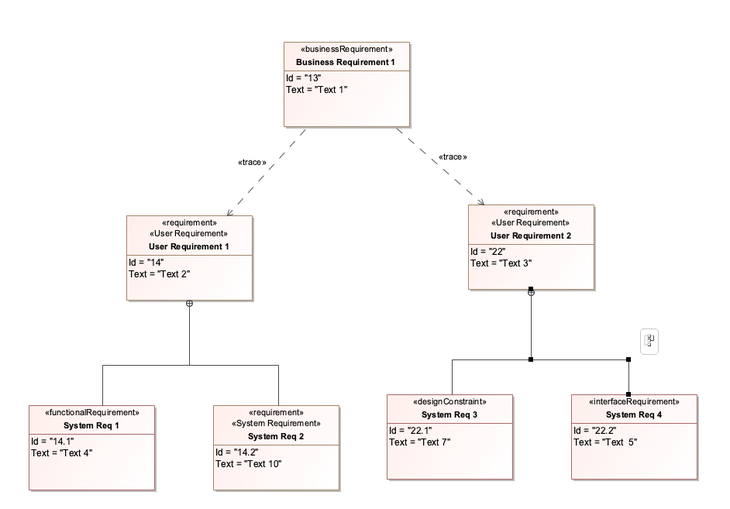
As you can see, specifications are depicted via these image blocks, and the relationships between various models are obvious.
This approach is a fantastic alternative to writing a specifications document, as the visualizations leave little room for doubt.
With this strategy, every employee will be able to read and understand the product’s requirements.
Set standards for writing clean code
Code is much easier to write when uniform.
Imagine if your codebase switched between Camel Case and Snake Case—developers would constantly mentally jump between the conventions, making the code itself difficult to navigate.
As such, it’s best to set standards for writing clean code, thus creating a more productive environment. There are even certain coding standards that immediately highlight software bugs.
Joel Spolsky knows this, urging developers to do the following:
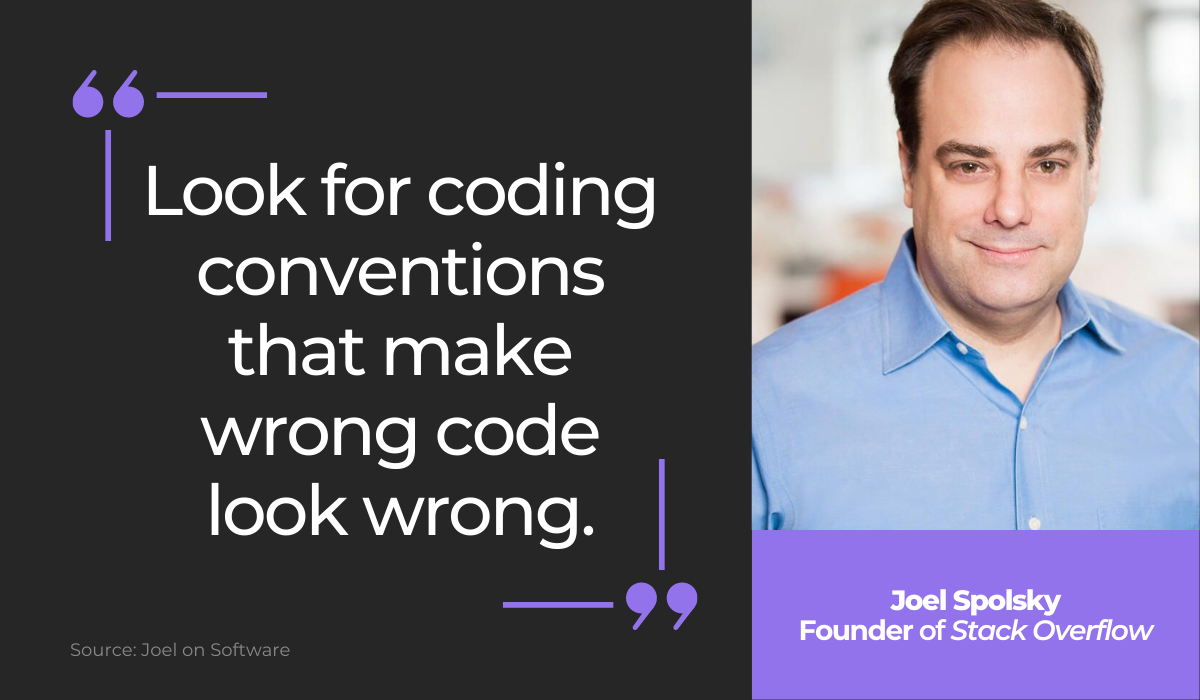
For example, the Linux kernel coding style demands the following indentation convention:

Although this might seem excessive, there’s solid reasoning behind it. With such large indentations, the code is easy to read if there are fewer than three indentation levels.
If you have four (or more) indentation levels, the code is difficult to view, which serves as a warning sign that the functions are nested too deep.
The developer will automatically see that the code is messy and rewrite it to be less complex, thus limiting possible errors.
This is a fairly universal standard. However, there are also language-specific practices for reducing errors. For embedded C, Michael Barr recommends:
Braces ({ }) shall always surround the blocks of code (also known as compound statements) following if, else, switch, while, do, and for keywords. Single statements and empty statements following these keywords shall also always be surrounded by braces.
Here’s this advice in practice:
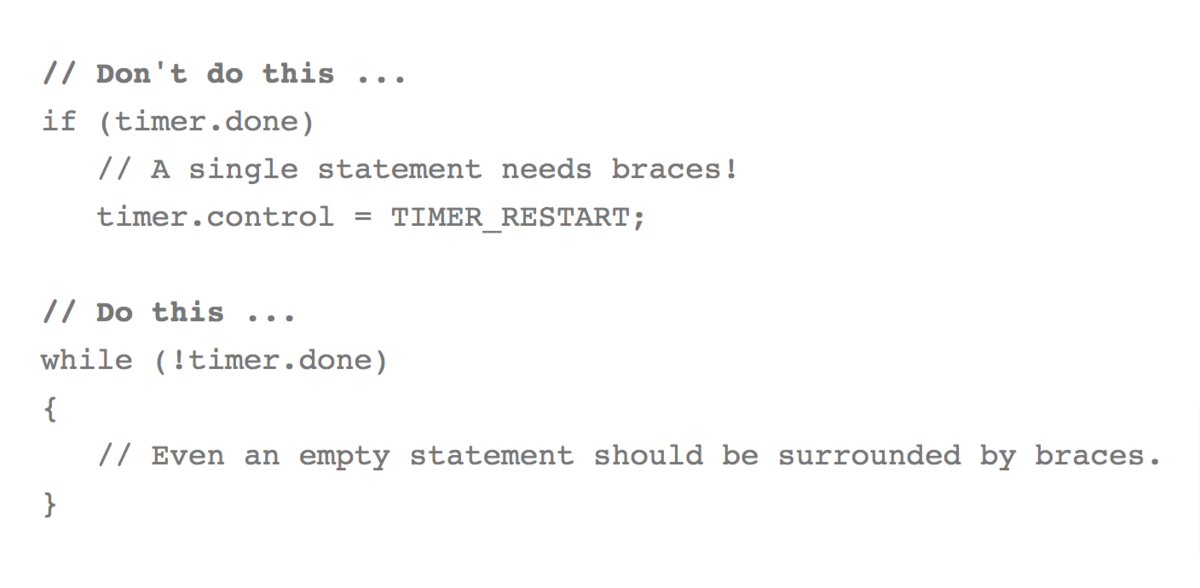
Omitting braces in such statements makes it difficult to maintain the code.
If nearby code is changed or commented out, and these conditional statements aren’t in braces, the edit will likely also impact the conditional.
Such slip-ups can then easily cause software bugs.
These seemingly harmless yet dangerous decisions aren’t limited to C—similar arbitrary mistakes can also cause problems in Java.
Generally speaking, == shouldn’t be used to compare strings in Java. This is because == actually compares the reference of the string, i.e., if they’re the same object.
Conversely, the equals() method compares if the value of the strings is equal.
Look at the following code:
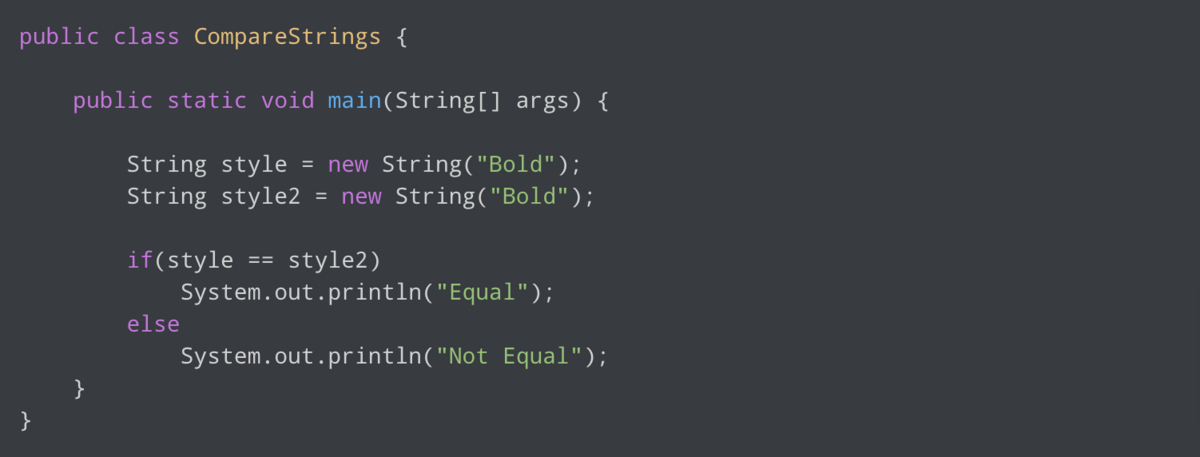
Its output is:

This is because the two string constructs are clearly not the same object.
However, if we employ the equals() method, as shown below:
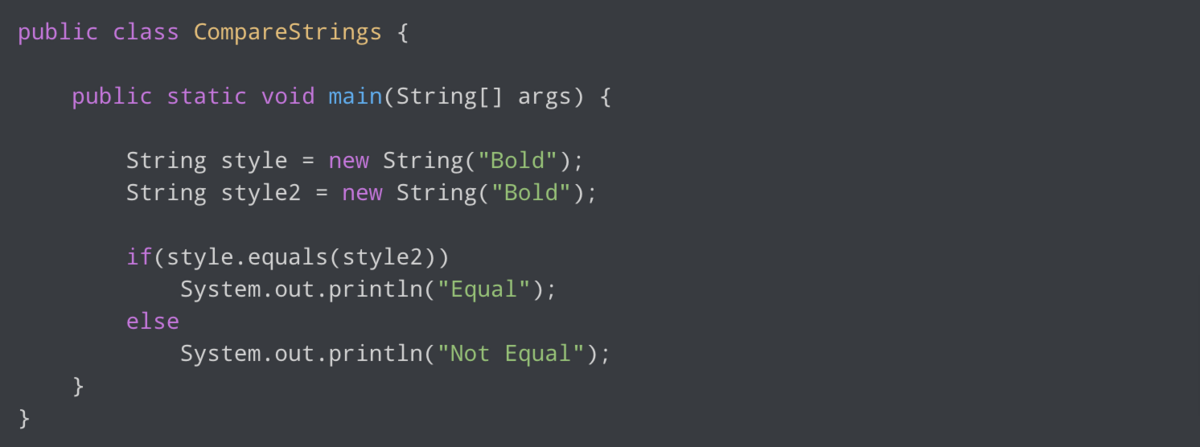
Its output is:

This is because their value is the same, as the equals() method indicates.
If your developers don’t know this distinction and use == to compare the value of strings, they can misunderstand and build code on faulty foundations.
Such constructions are a volatile breeding ground for bugs.
Given these dangers, it’s best to clearly define coding standards for general practices (indentation, DRY, KISS, etc.) and language-specific conventions.
Doing so should significantly prevent software bugs.
Use test-driven development
Test-driven development is an approach that flips standard software development on its head. In traditional Waterfall workflows, development precedes testing—the code is always written first.
However, with test-driven development, it’s the other way around—the tests are written first.
These tests define the software’s exact functionalities and therefore establish its necessary operations.
If the code that is to be written passes all tests, you’ll have confirmation the software is working perfectly.
But if any tests fail, it’s an obvious sign improvement is necessary. The software’s required quality level is immediately pre-defined with these tests.
Such an approach significantly aids in preventing software bugs, as the pre-written tests will detect any errors right at the beginning.
Test-driven development consists of three steps, called the Red-Green-Refactor cycle. This process is displayed below:
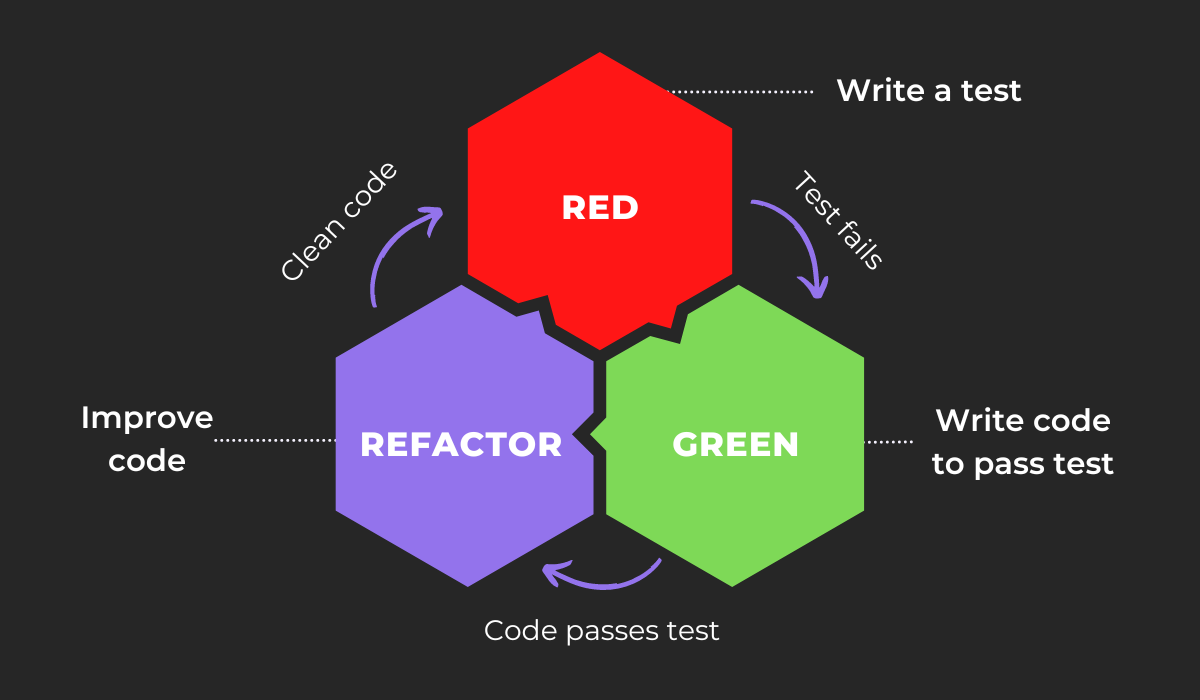
Once a test is written, it must always fail first, simply because there’s no code to activate it. Then the developers write the code, striving to make it pass the tests.
After that’s done and the code passes, they refactor the code to make it as clean and readable as possible.
The thoroughness this approach provides is an excellent asset for reducing software bugs. Authors Maria Siniaalto and Pekka Abrahamsson had the same observation in their study:
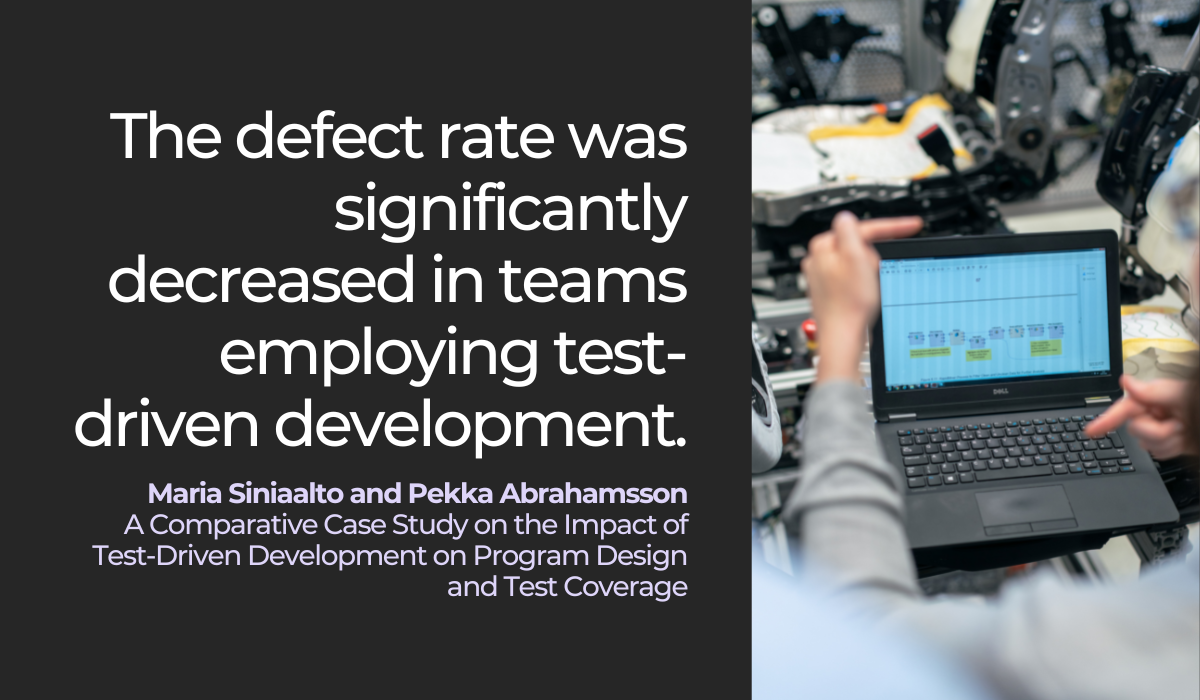
The two developers noticed a substantial error reduction in teams employing test-driven development.
Furthermore, debugging efforts were faster with those organizations that used test-driven development.
Their research isn’t the only investigation to uncover such results. In a joint Microsoft and IBM effort, researchers discovered the following:
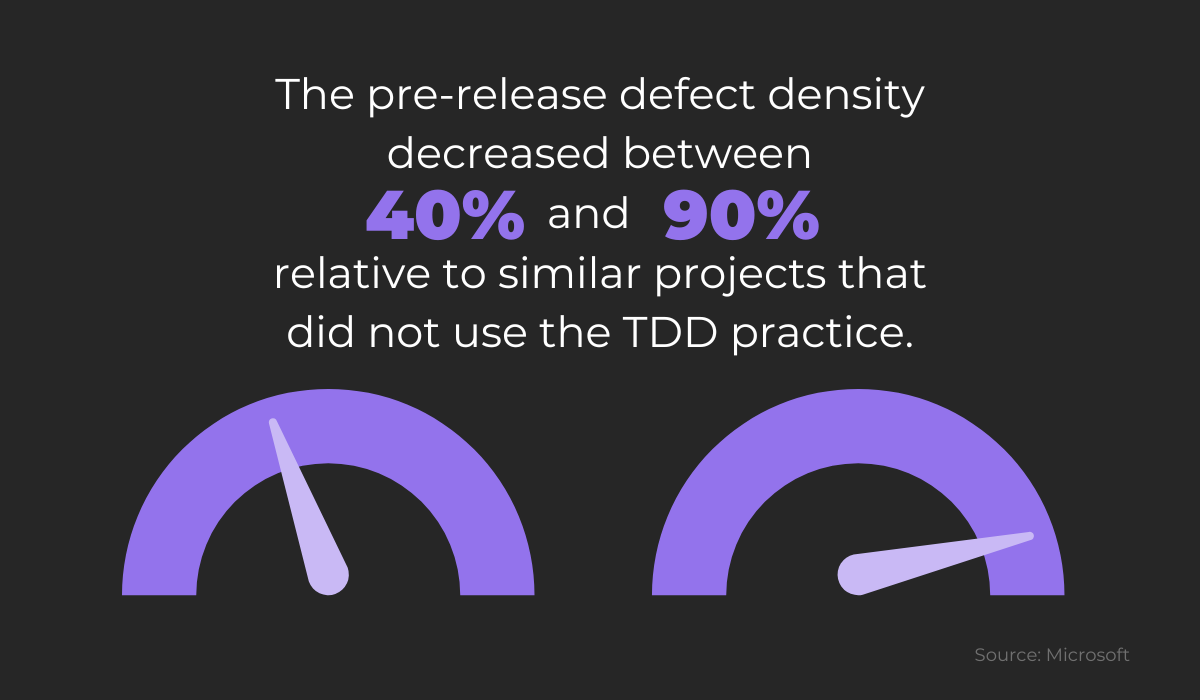
When using test-driven development, IBM experienced a 40% drop in the number of software bugs, whereas Microsoft measured between 60% and 90%.
The dramatic bug reduction rate results in cleaner, more stable code.
It’s clear that test-driven development is well worth implementing to keep your software error-free. That being said, this practice is Agile, so it’s best to take an iterative approach.
In other words, smaller, focused tests are preferable to larger tests with a wider scope.
Three minor tests surveying 30 lines of code will yield better, more detailed results than one major test surveying 100 lines.
Ben Miller explains the concept further:
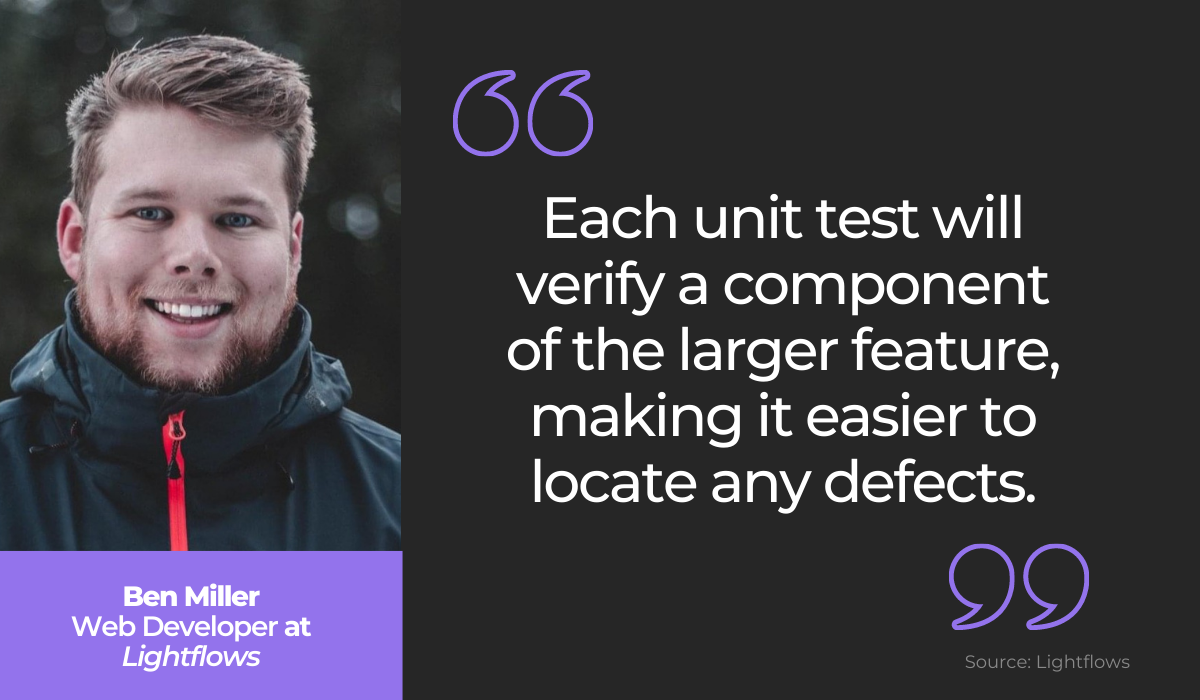
Since each smaller test verifies sections of a larger whole, your QA testers will have a more complete and detailed understanding of the codebase’s functionality.
This approach is a cornerstone of test-driven development and is a surefire method to prevent software bugs from appearing.
Consider behavior-driven development
Behavior-driven development is an excellent complement to test-driven development (or as a stand-alone practice).
This methodology works to bridge the communication gap between technical and non-technical employees.
The chief benefit of this approach is its use of a common domain-specific language, which adapts natural languages (e.g., English) into test scripts.
This makes tests accessible and understood by all project participants, not just the technical team.
This heightened insight into tests removes most communication barriers and therefore reduces possible bugs.
Everyone is on the same page, meaning everyone can create and review test cases and spot potential pitfalls.
When mentioning everyone, we’re referring to the principal players of behavior-driven development, described below:
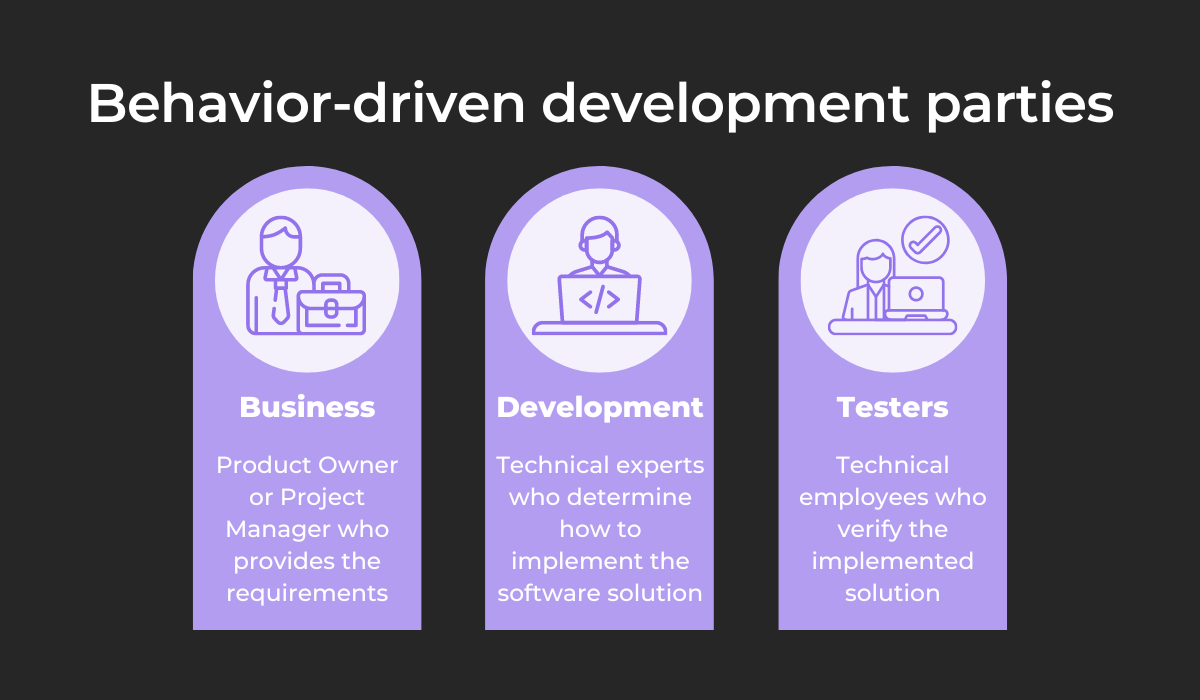
The business team usually consists of the Product Owner, Project Manager, and stakeholders. They provide the software requirements, detailing the necessary software solution.
Then, the development team (i.e., you and your developers) must determine how to implement this solution.
Finally, the QA testers will verify that the development team’s solution is functioning correctly.
Of the three groups, the developers typically have the most technical knowledge, whereas the business side has the least.
Ideally, these three departments will meet at the beginning of a project and discuss the scenarios and domains to be enacted, especially regarding the tests.
Development should then progress as follows:
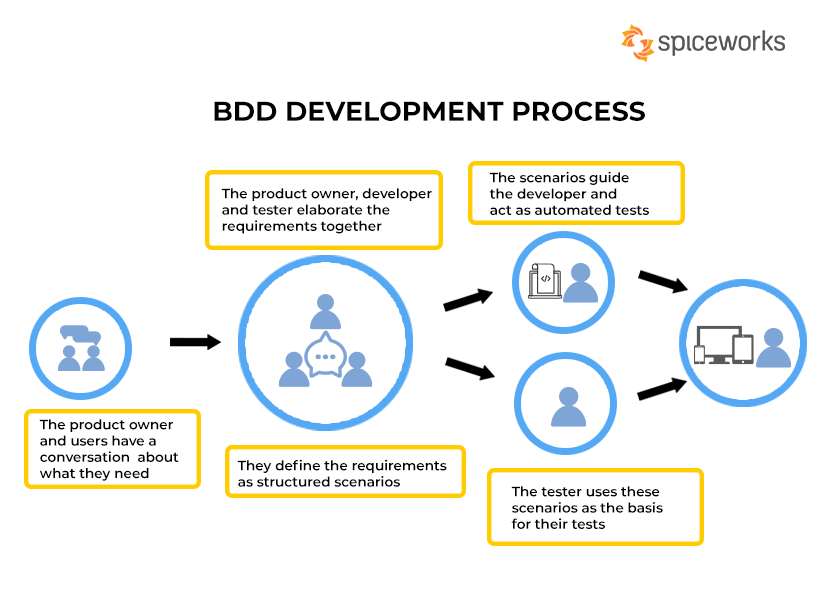
With such transparent, direct collaboration from all parties, there’s not much chance of misunderstandings and miscommunication.
After all, everyone agrees on the requirements, software, and testing scenarios, leaving little room for error.
Dave Wade-Stein commented on this as well, highlighting the importance of cooperation:
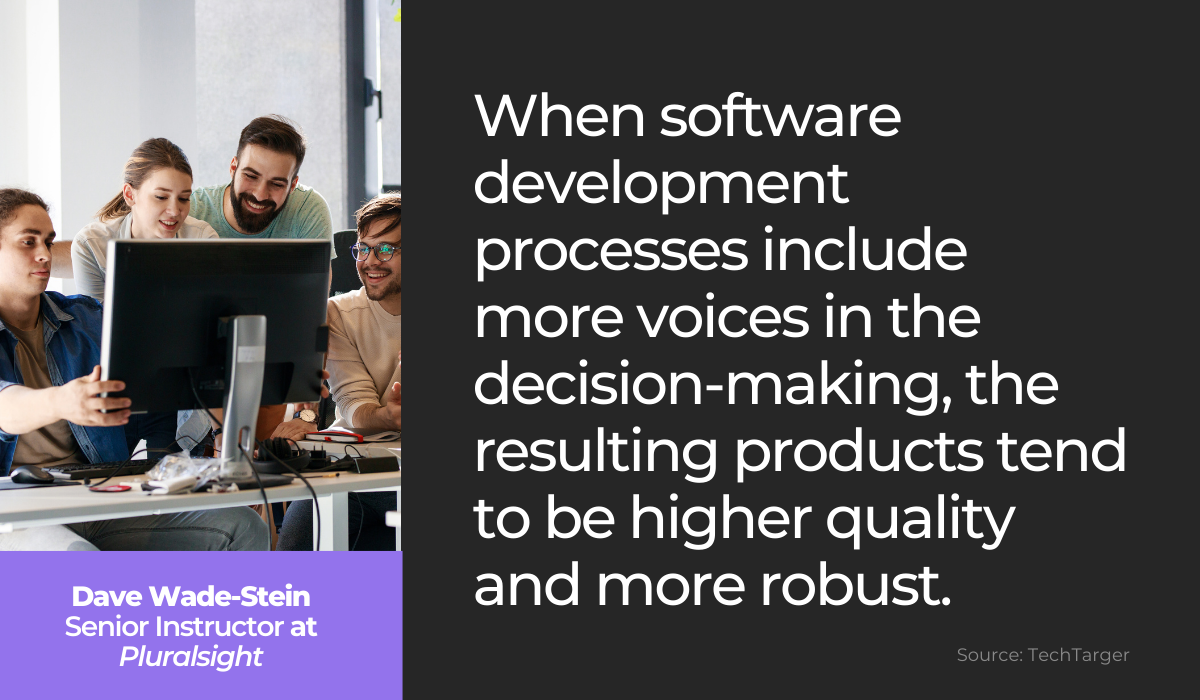
With everyone aligned and contributing to the software’s design right from the beginning, there’s a far greater chance of a high-quality, bug-free product.
However, behavior-driven development’s greatest asset is utilizing the English language for testing instead of script-based testing.
There are many online tools that can help you out with this, such as Testsigma.
With Testsigma, users can create test steps for any scenario by simply writing them in standard English.
There is absolutely no code required, which allows everyone—even those without technical knowledge—to review test cases and participate in testing.
Here’s a sample test:
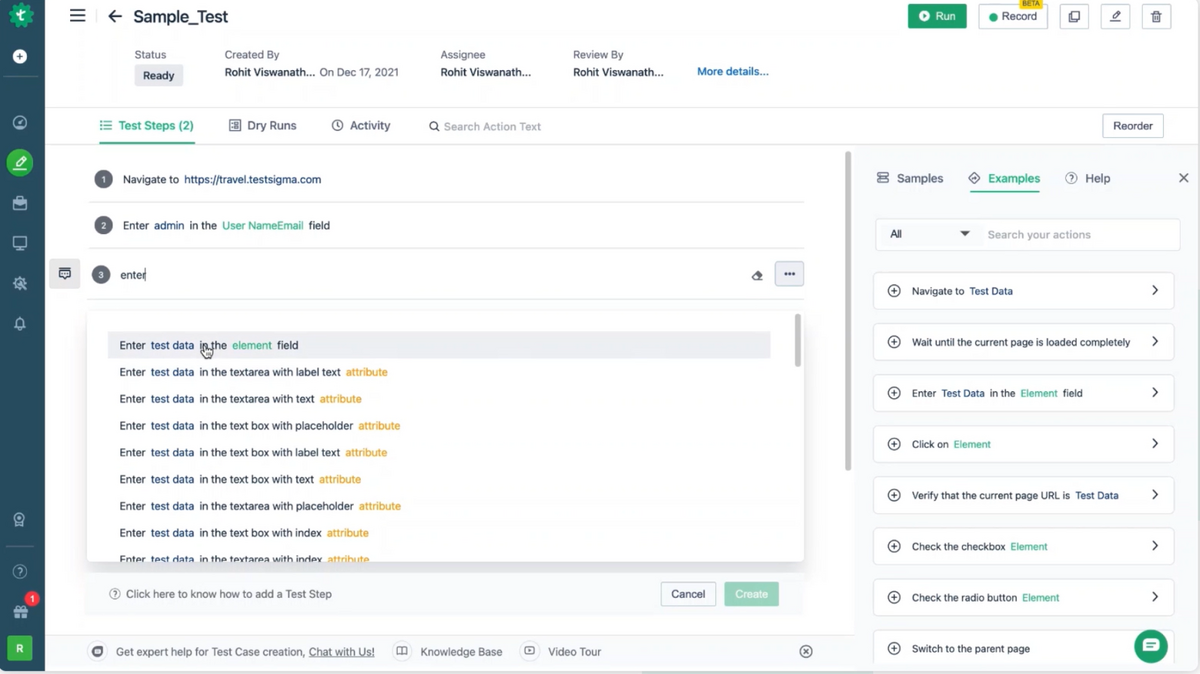
As you can see, the test steps are expressed in direct, plain English.
Such an approach removes any communication silos, and everyone can make testing suggestions, thus greatly diminishing possible software bugs.
Focus on continuous integration
Merging code is often a gamble, as it’s always possible the code changes will clash with the existing code.
However, continuous integration offers a solution. The practice encourages developers to commit small code changes very frequently (sometimes even daily).
Once the code is merged, continuous integration validates it by automatically building the application and running automatic testing to ensure there’s no incompatibility.
For example, here’s a sample CI/CD pipeline:
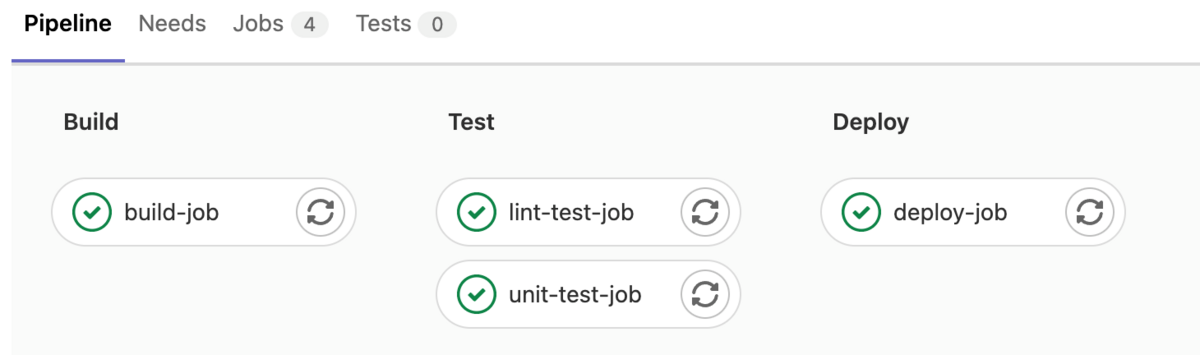
This pipeline contains four jobs: one for building, one for deploying, and two for testing. All jobs will be automatically executed once the code changes are committed, including the testing.
Such automated testing will immediately locate any conflicts and pinpoint bugs in the making.
If the automated tests fail, this is actually a desirable outcome—they’re preventing errors from entering the main code branch.
One of the principal maxims of continuous integration is: Fail fast, fail often. The saying encourages failure, as test failure is an opportunity to correct defects.
Jonathon Hensley has also commented on this concept:
Failure is not the objective of any organization, but to learn is critical to every organization. And so we have to get Fail fast and fail often as a mechanism for learning.
If your tests fail, this is a blessing in disguise. You’ll be able to prevent bugs from infiltrating the codebase, which is preferable to debugging in production.
However, to fully embrace the Fail first, fail often approach of continuous integration, you’ll first need to set up testing automation. Luckily, there are plenty of online tools to assist you.
For example, Katalon Studio is a versatile automation testing tool optimized for continuous integration.
The resource boasts rich integration options with CI/CD tools. Here’s an overview from their documentation:
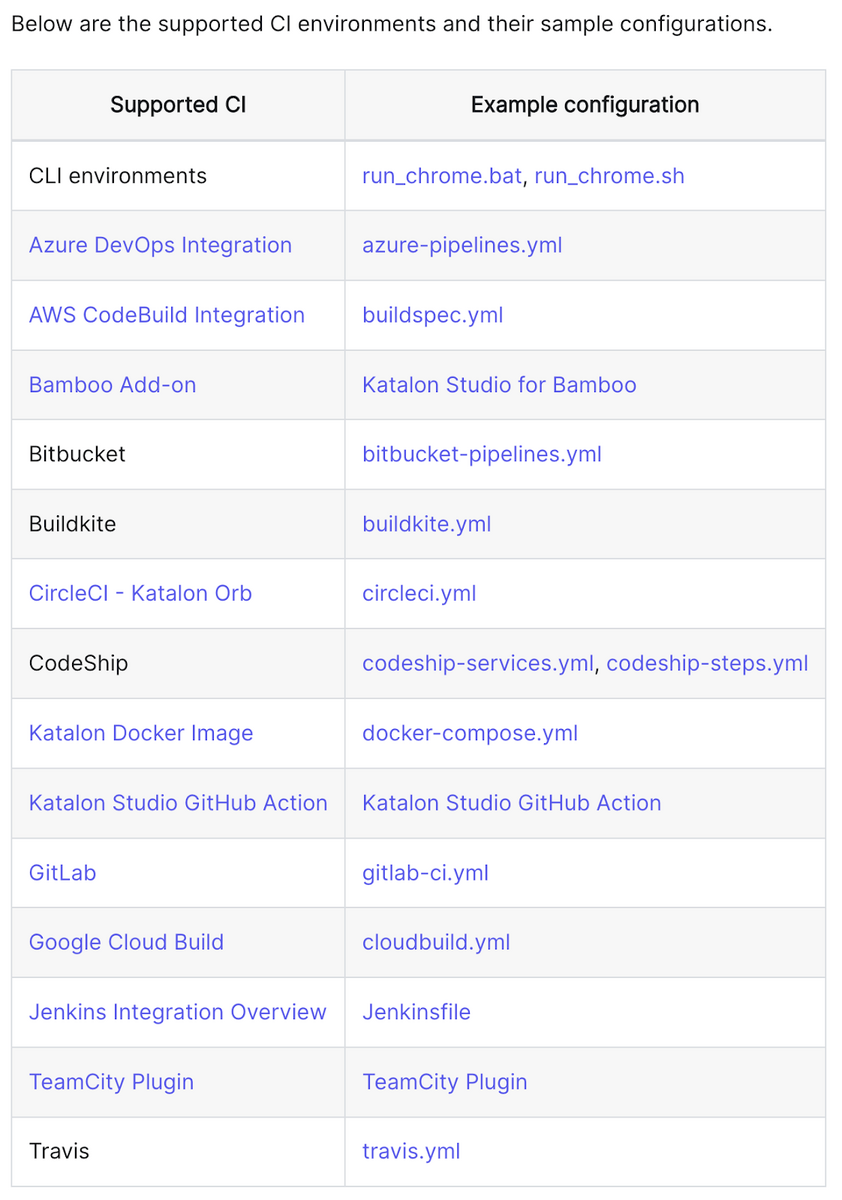
The tool easily integrates with several popular CI/CD tools, such as Jenkins, Travis CI, TeamCity, and more.
If you have an automation testing tool that’s easily integrated with these resources, you’re well on your way to preventing software bugs.
In case you don’t have such an automation testing tool, don’t worry. Jenkins is an open-source CI/CD tool that boasts over 1,800 plugins.
At least one of those options should meet your testing automation needs.
To facilitate this process, Jenkins is equipped with a test harness, described below:
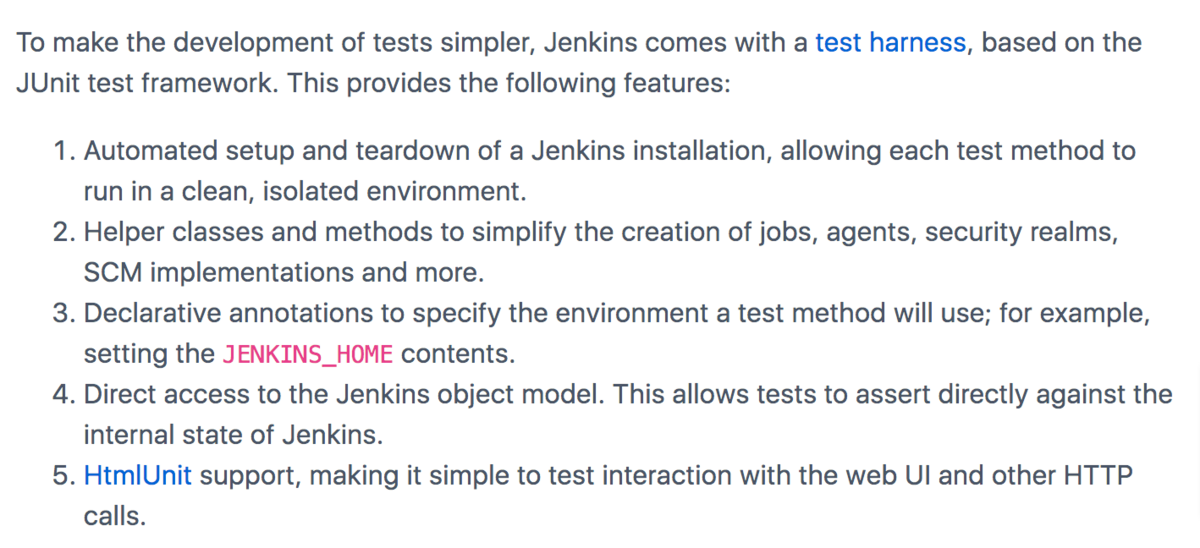
With this test harness, testing in Jenkins is significantly more manageable, as all possible plugins should automatically function seamlessly with the tool.
Developing tests will be a walk in the park, and you’ll dramatically reduce software bugs in your codebase.
Conclusion
Although effective debugging will quickly remove defects from your codebase, it’s preferable that bugs aren’t there in the first place. There are several tactics to accomplish this.
For starters, bugs are less likely to occur if your team is clear about product requirements and sets standards for writing clean code.
It’s also well worth utilizing test-driven development or behavior-driven development—or both!
Finally, you’d do well to focus on continuous integration, to automate testing cycles, and therefore reduce the number of software errors.
Follow these practices, and you should soon see a dramatic reduction in the occurrence of bugs in your product.